Problem Description
Place elements organized in an array or list in either ascending or descending order.
Algorithm Description
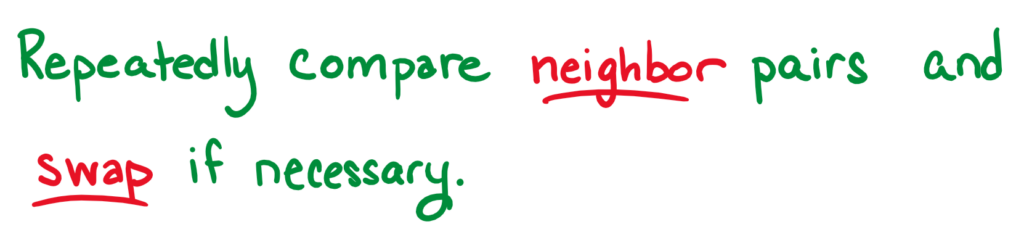
Bubble sort is a simple sorting algorithm that repeatedly steps through the list or array to be sorted, compares each pair of adjacent items, and swaps them if they are in the wrong order.
Videos
Going through Bubble Sort with Actual Input
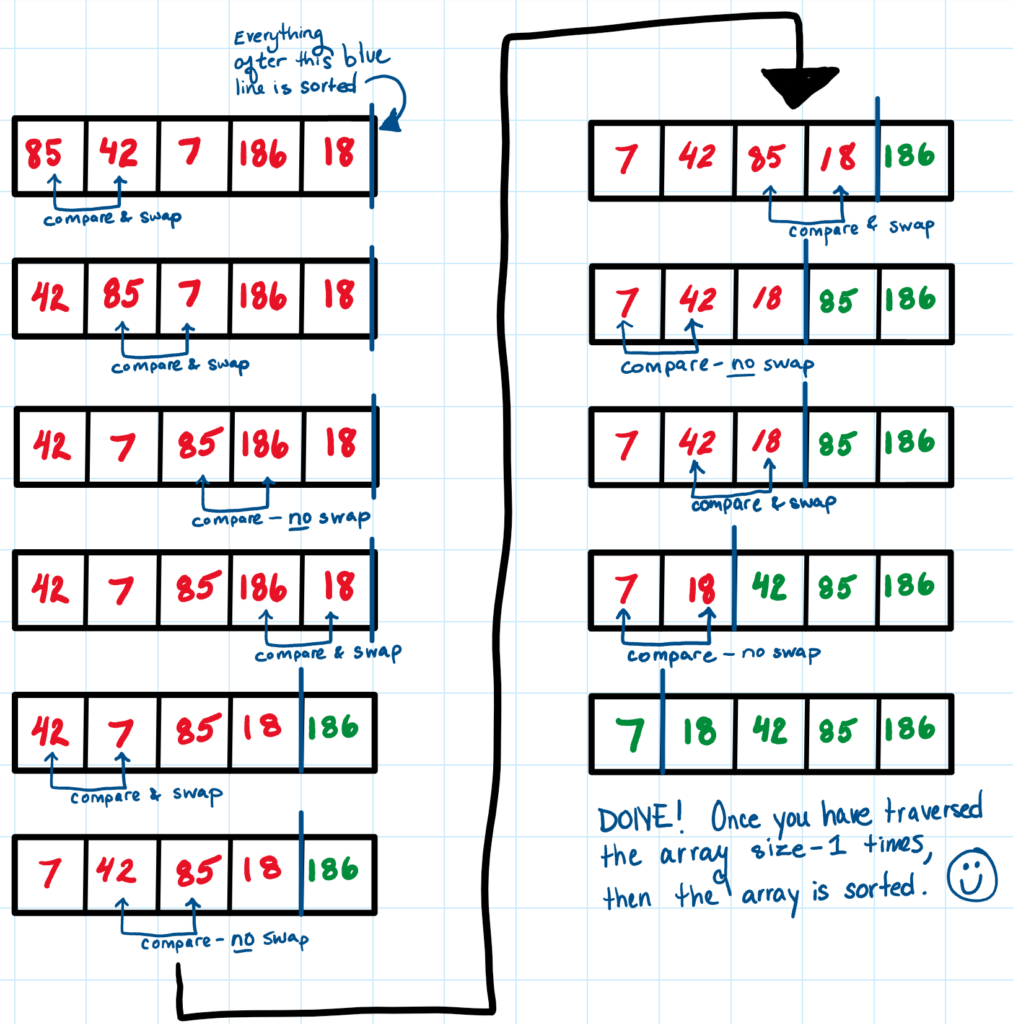
C++ Code
#include <iostream>
using namespace std;
void displayArray(int*, int);
void bubbleSort(int*, int);
int main(){
int myArr[5] = {85, 42, 7, 186, 18};
displayArray(myArr, 5);
bubbleSort(myArr, 5);
displayArray(myArr, 5);
return 0;
}
void bubbleSort(int *arr, int size){
int tempForSwap;
cout << "\nSorting the array with the Bubble Sort algorithm.\n\n";
//maxElement will hold the subscript of the last element
//that is to be compared to its immediate neighbor
for(int maxElement=(size-1); maxElement > 0; maxElement--){
for(int i=0; i<maxElement; i++) {
if(arr[i] > arr[i+1]) { //swap!
tempForSwap = arr[i];
arr[i] = arr[i+1];
arr[i+1] = tempForSwap;
}
}
}
}
void displayArray(int *arr, int size){
cout << "\n--------------------The array: ";
for(int i=0; i<size; i++){
cout << arr[i] << " ";
}
cout << "--------------------\n";
}
Other Resources
- GeeksforGeeks: https://www.geeksforgeeks.org/bubble-sort
- Programiz: https://www.programiz.com/dsa/bubble-sort
- Visualgo (Visualization of Bubble Sort): https://visualgo.net/bn/sorting?slide=6